Type Qualifiers in C
In C programming language, type qualifiers are the keywords used to modify the properties of variables. Using type qualifiers, we can change the properties of variables. The c programming language provides two type qualifiers and they are as follows...
- const
- volatile
const type qualifier in C
The const type qualifier is used to create constant variables. When a variable is created with const keyword, the value of that variable can't be changed once it is defined. That means once a value is assigned to a constant variable, that value is fixed and cannot be changed throughout the program.
The keyword const is used at the time of variable declaration. We use the following syntax to create constant variable using const keyword.
const datatype variableName ;
When a variable is created with const keyword it becomes a constant variable. The value of the constant variable can't be changed once it is defined. The following program generates error message because we try to change the value of constant variable x.
Example Program
#include<stdio.h>
#include<conio.h>
void main(){
int i = 9 ;
const int x = 10 ;
clrscr() ;
i = 15 ;
x = 100 ; // creates an error
printf("i = %d\nx = %d", i, x ) ;
}
Output:
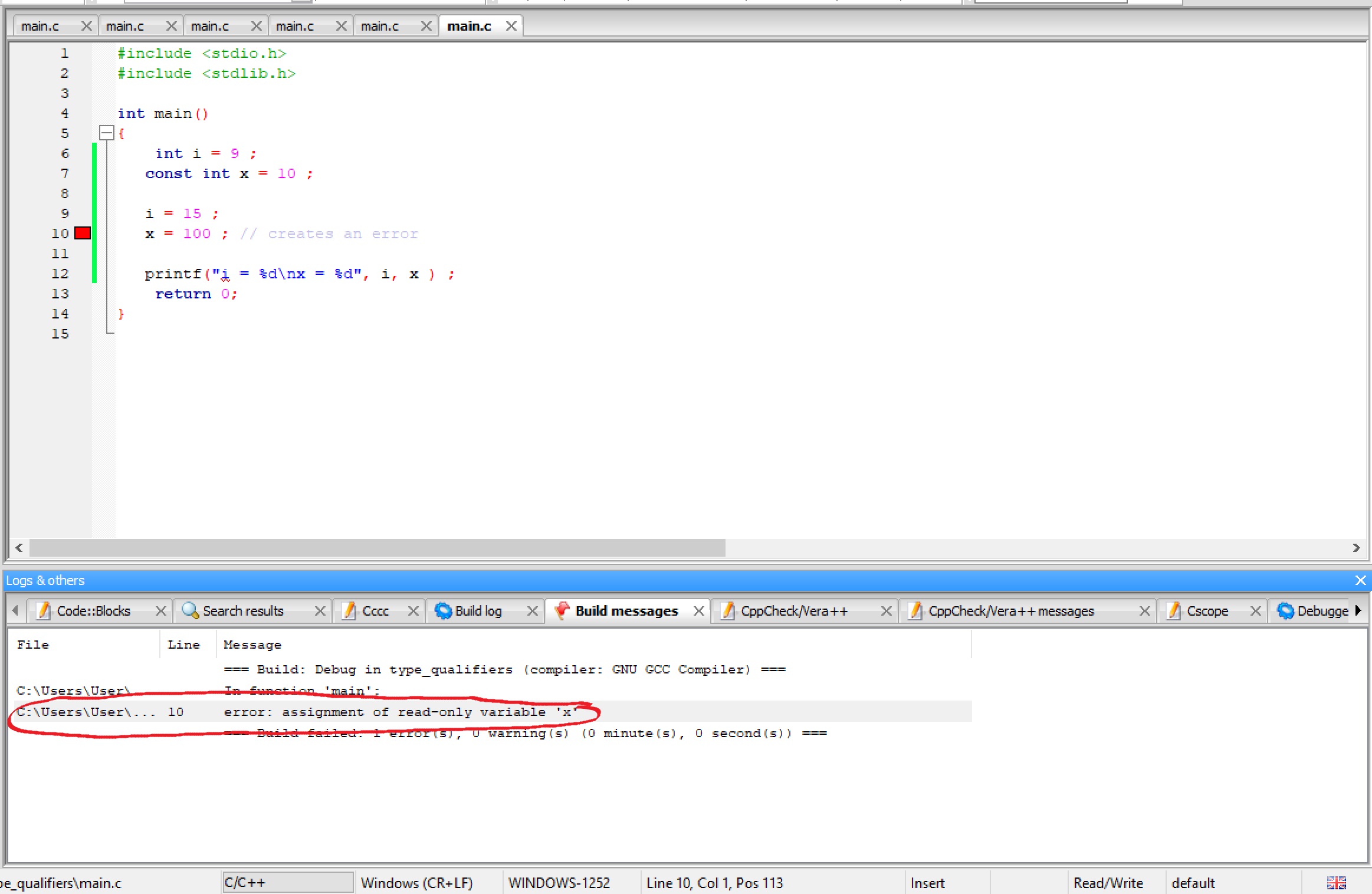
→ For more details of const keyword and constant variables in C refer to C Constants.
volatile type qualifier in C
The volatile type qualifier is used to create variables whose values can't be changed in the program explicitly but can be changed by any external device or hardware.
For example, the variable which is used to store system clock is defined as a volatile variable. The value of this variable is not changed explicitly in the program but is changed by the clock routine of the operating system.